Do you want to know how to use the ftrack API? You’re in the right place. Below, we run through setting up the ftrack API and putting it into action.
Very rarely are two creative pipelines the same—which is why we built the ftrack API. With the ftrack API, you can customize ftrack Studio to work the way you need it and deliver projects in sync with your unique studio workflows.
For example, say there’s a specific platform you’d love to integrate with ftrack Studio, but it’s not yet on ftrack’s integrations list. Or maybe you want to create something particular to your studio hiring process, like setting up a studio-specific start-up wizard for new starters. Or perhaps you’re just fed up with spending a lot of time on tasks that could be much more efficiently batch-automated. The ftrack Python API can help you achieve these things and much more.
If you’re interested in trying out the ftrack API, but you’re not sure where to start, then you’re in the right place. Below, you can follow our guide to getting started with the ftrack API and access some useful links to get you off the ground.
Before we dive in, check out how others are using and benefiting from customizing ftrack Studio and bringing the platform in line with their unique studio requirements. Head to the end of the blog to see a recent webinar about the ftrack API, too!
A guide to getting started with the ftrack API
ftrack is primarily a web application with an intuitive, modern interface, which lets users interact with creative projects and their assets while in production. If you want to dig deeper and start automating processes or get creative with how you use your data, you’ll want to roll up your sleeves and dive into the power of the ftrack API.
Below, we’ll run through the steps to getting started with ftrack’s Python API. We’ll use Python in this example due to its ease of use and readability (both being reasons for Python being an industry-standard programming language).
If you get stuck at all, you can find plenty of additional support in the form of documentation and ftrack’s community support. Also, be sure to check out our recent ftrack API 2.0 update.
Here’s the best starting point for using the ftrack Python API: ftrack-python-api.readthedocs.io/en/stable
1. Install Python, if you haven’t already
If you don’t have Python installed, then your first step is to install it. There’s a good chance your system already includes Python, but if it doesn’t then you can get it from python.org. In this post, we’ll be using Python 3.8.
Once installed, verify you have Python working with:
$ python —version python 3.8.9
2. Set up a virtual environment for experimentation (if you want to!)
One more nice-to-have tool when working with Python is a virtual environment, created using Python’s venv module. Virtual environments allow you to set up a sandbox where you can specify Python versions for use and install Python modules without polluting your system’s Python environment. You can create and “activate” a virtual environment with the following commands:
$ python3 -m venv /path/to/new/virtual/environment $ cd /path/to/new/virtual/environment $ source ./bin/activate
Please note that this is our suggestion/best practice approach to working with Python via virtual environments. Other options are available, but we’ll stick with this approach in this tutorial.
3. Install the ftrack API and iPython
Now we can install the ftrack API using PIP (the Python package installer). Here’s what to run:
$ pip install ftrack-python-api […some output omitted…] Successfully installed ftrack-python-api-2.3.0
Another helpful module that’s especially useful when exploring new APIs is iPython. iPython is an enhanced interactive Python shell that helps you make the most of using Python interactively. The most applicable features include:
- Comprehensive object introspection.
- Input history, persistent across sessions.
- Extensible tab completion, with support by default for completion of python variables and keywords, filenames, and function keywords.
Note that iPython is not required, but it’s really nice to have as you explore the API interactively. Install iPython with:
$ pip install ipython
Then, start an ipython shell with the following command:
% ipython Python 3.8.9 (default, Oct 26 2021, 07:25:54) Type 'copyright', 'credits' or 'license' for more information IPython 8.0.0 -- An enhanced Interactive Python. Type '?' for help. In [1]:
4. Get API authentication credentials
One more thing you’ll need is API authentication credentials. You can get API authentication credentials from your account page in ftrack Studio. To do so, head to your My Account page. You’ll see a “Personal API Key”, your “Username” (email), and the base URL for your ftrack instance from your browser’s address bar. Once you have these credentials, you can configure them by setting the following environment variables for your shell (bash, zsh):
- FTRACK_SERVER=”https://mycompany.ftrackapp.com”
- FTRACK_API_USER=”rob.orsini@ftrack.com”
- FTRACK_API_KEY=”7545384e-a653-11e1-a82c-f22c11dd25eq”
Alternatively, you can enter your credential in code when you create your Session object (which you’ll use to interact with the API). Here’s how that looks:
In [1]: import ftrack_api In [2]: session = ftrack_api.Session( ...: server_url="https://mycompany.ftrackapp.com", ...: api_key="7545384e-a653-11e1-a82c-f22c11dd25eq", ...: api_user="rob.orsini@ftrack.com", ...: )
Or, if you’ve configured your credentials using environment variables, then the commands would be:
In [1]: import ftrack_api In [2]: session = ftrack_api.Session()
Notice in the examples above that we first imported the ftrack API package. We then configured and instantiated a Session object. If both of these commands run without errors, then you’re ready to get exploring!
All communication with an ftrack server takes place through the Session object. To learn more about how this object works, see this documentation on understanding sessions.
5. Explore your project data
Ideally, your API credentials will allow you to read and write data to your server. While both are important, it is easiest to explore in read-only mode. So let’s write our first query and have a look around. But first, let’s take a look at all the “types” in our database. To do so, type the following in your iPython session:
In [3]: session.types.keys() Out[3]: dict_keys(['Asset', 'Milestone', 'Task', 'Project', 'Note', ‘Shot', ‘Sequence'])
You’ll see a much longer list of types above, but the list is reduced here for the sake of simplicity.
At this point, be sure to familiarize yourself with ftrack’s excellent API reference, which is available in the Help section (top right corner) of your ftrack instance. You can always refer back to this resource as you explore using the API.
Let’s start with the Project type. Here’s a query to list all ftrack Studio projects:
In [4]: session.query("Project").all() Out[4]: [<dynamic ftrack Project object 4569254112>, <dynamic ftrack Project object 4568971584>, <dynamic ftrack Project object 4568970912>]
Better yet, let’s iterate over the results of this query by printing the “name” of each project in the results set with:
In [5]: projects = session.query(“Project”).all() In [6]: [p["name"] for p in projects] Out[6]: [‘Demo Project’, ‘Avengers Movie', ‘Fender Commercial']
Let’s take the first project and explore some of its attributes and the entities they point to and continue down into the object tree as we explore:
In [7]: demo_project = projects[0] Out[7]: [('created_at', <Arrow [2020-05-19T10:19:07+00:00]>), ('managers', <ftrack_api.collection.Collection at 0x110648c70>), ('calendar_events', <ftrack_api.collection.Collection at 0x110557130>), ('color', '#FFEB3B'), ('_link', [{'type': 'Project', 'id': '2c5fc83e-99ba-11ea-9970-ca9d6ff8834e', 'name': ‘Demo Project'}]), ('full_name', 'Demo Project'), ('disk', <dynamic ftrack Disk object 4936562864>), ('children', <ftrack_api.collection.Collection at 0x1105d1f70>), ('timelogs', <ftrack_api.collection.Collection at 0x1263dfc70>), ('end_date', <Arrow [2021-12-31T00:00:00+00:00]>), ('parent_id', None), ('created_by', <dynamic ftrack User object 4936948464>), […some output omitted…]
Now we see all the top-level attributes of our project and their respective types. We can access each of these attributes, and based on each subsequent entity type we can inspect or iterate down through the project’s hierarchy. For example, to see how many “Calendar Events” we have in the Demo Project, you can simply do this:
In [8]: len(demo_project[“calendar_events"]) Out[8]: 49
Once you’re comfortable querying for things (“reading”), then you can move on to modifying and creating things (“writing”). For that, we’ll direct you toward the API documentation. You can find a variety of links below to help you with your research.
Helpful resources
Explore the ftrack API
Start your free ftrack Studio trial to see how the ftrack API can support your next project.
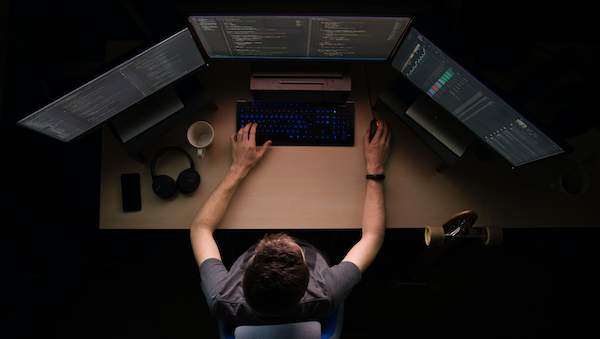
More from the blog

Enhanced performance in ftrack Studio: Fine-tuning for speed, reliability, and security
Chris McMahon | API, Developer, New features, Product, Productivity, Studio | No Comments
Backlight and the Visual Effects Society forge a partnership for the VES Awards judging process
Kelly Messori | Case Study | No Comments
Presenting the new sidebar: Enhancing project navigation in ftrack Studio
Chris McMahon | New features, Product, Release, Studio | No Comments
Achieving Better Feedback Cycles and Faster Nuke Workflows at D-Facto Motion Studio
Kelly Messori | Case Study, Studio | No Comments
Making the switch: The transition to cineSync 5
Mahey | Announcements, cineSync, News, Product | No Comments
Supporting Your Studio: Free ftrack Studio Training and Office Hours from Backlight
Kelly Messori | News | No Comments
What’s new in cineSync – a deeper iconik integration, laser tool, OTIOZ support, and more
Chris McMahon | cineSync, New features, Product, Release | No Comments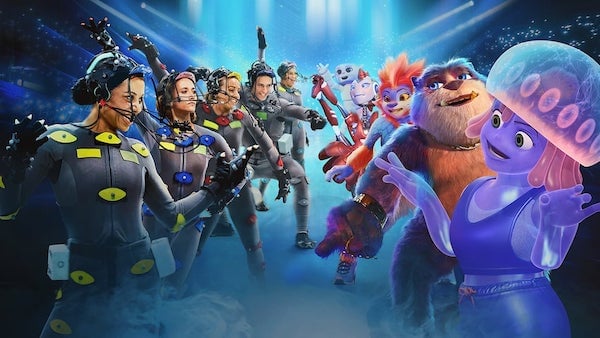